Java is a programming language and a platform. Java is a high level, robust, object-oriented and secure programming language.
Our core Java programming tutorial is designed for students and working professionals. Java is an object-oriented, class-based, concurrent, secured and general-purpose computer-programming language. It is a widely used robust technology.
What is Java?
Java is a programming language and a platform. Java is a high level, robust, object-oriented and secure programming language.
Java was developed by Sun Microsystems (which is now the subsidiary of Oracle) in the year 1995. James Gosling is known as the father of Java. Before Java, its name was Oak. Since Oak was already a registered company, so James Gosling and his team changed the name from Oak to Java.
Platform: Any hardware or software environment in which a program runs, is known as a platform. Since Java has a runtime environment (JRE) and API, it is called a platform.
Application
According to Sun, 3 billion devices run Java. There are many devices where Java is currently used. Some of them are as follows:
- Desktop Applications such as acrobat reader, media player, antivirus, etc.
- Web Applications such as irctc.co.in, javatpoint.com, etc.
- Enterprise Applications such as banking applications.
- Mobile
- Embedded System
- Smart Card
- Robotics
- Games, etc.
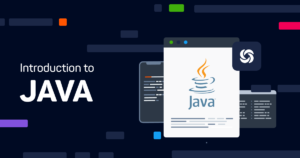
Java Course Syllabus:-
Introduction
- Why Java
- Paradigms
- Diff B/W Java & Other (C,C++)
- Java History
- Java Features
- Java programming format
- Java Statements
- Java Data Types
OOPS (Object Oriented Programming & Systems)
- Introduction
- Object
- Constructors
- This Key Word
- Inheritance
- Super Key Word
- Polymorphism (Over Loading & Over Riding)
- Abstraction
- Interface
- Encapsulation
- Introduction to all predefined packages
- User Defined Packages
- Access Specifiers
STRING Manipulation
Array
- What is Array
- Single Dimensional Array
- Multi-Dimensional Array
- Sorting of Arrays
Packages Exception Handling
- Introduction
- Pre-Defined Exceptions
- Try-Catch-Finally
- Throws, throw
- User Defined Exception examples
I/O Streams
- Introduction
- Byte-oriented streams
- Character – oriented streams
- File
Multithreading
- Introduction
- Thread Creations
- Thread Life Cycle
- Life Cycle Methods
- Synchronization
- Wait() notify() notify all() methods
Wrapper Classes
- Introduction
- Byte, Short, Integer, Long, Float, Double, Character
- Boolean classes
Inner Classes
- Introduction
- Member Inner Class
- Static Inner Class
- Local Inner Class
- Anonymous Inner Class
Collection Frame Work
- Introduction
- Util Package interfaces, List, Set, Map
- List Interface 7 Its Classes
- Set Interface & Its Classes
- Map Interface & Is Classes
AWT
- Introduction
- Components
- Event-Delegation-Model
- Listeners
- Layouts
- Individual Components Lable, Button, Check Box, Radio Button,
- Choice, List, Menu, Text Field, Text Area
SWING (JFC)
- Introduction Diff B/W AWT and SWING
- Components hierarchy
- Panes
- Individual Swings components J Label
- JButton, JTextField, JTextAres
Advanced Java Syllabus (J2EE Course Syllabus)
Introduction to Enterprise Edition
- Distributed Multitier Applications
- J2EE Containers
- Web Services Support
- Packaging Applications
- J2EE 1.4 APIs
Web Server and Application Server
- Tomcat-Introduction
- Overview, installation, Configuring Tomcat
- Jboss server-Introduction
- Overview,installation and Configuration
- Comparison
SQL
JDBC
- Introduction
- JDBC Architecture
- Types of Drivers
- Statement
- Result Set
- Read Only Result Set
- Updatable Result Set
- Forward Only Result Set
- Scrollable Result Set
- Prepared Statement
Servlets
- Introduction
- Web application Architecture
- HTTP Protocol & HTTP Methods
- Web Server & Web Container
- Servlet Interface
- HTTPServlet
- GenericServlet
- Servlet Life Cycle
- Servlet Config
- Servlet Context
- Servlet Communication
- Servlet-Browser Communication
- sendError
- setHeader
- sendRedirect
- Web-Component Communication
- Servlet-Applet Communication
- Session Tracking Mechanisms
- Http Session
- Cookies
- URL-Rewriting
- Hidden-Form Fields
13.Filters&Wrappers
14.Listeners
15 Web-Security
JSP
- Introduction
- Jsp LifeCycle
- Jsp Implicit Objects & Scopes
- Jsp Directives
Jsp Scripting Elements
- declaratives
- scriptlets
- expressions
JSP Actions
Standard Actions
- useBean tag
- setProperty tag
- getProperty tag
- include tag
- forward tag
- param tag
- plug-in tag
- params tag
- fallback tag
- directives tag
- scriptlet tag
- expression tag
Custom Actions
JSTL & Tag Library
Download the Syllabus